アクセス数 累計:000,136,192 昨日:000,000,139 本日:000,000,026
|
|
|
|
【Amazon ランキング:DVD - 海外映画】
|
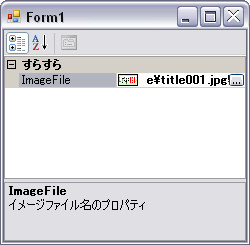 |
前回ファイル名を指定できるプロパティの作り方を紹介しましたが、今回はさらに進めてプロパティにプレビューを表示する機能をつけてみようと思います。
これはBackgoundImageプロパティ等でおなじみですが、イメージだけでなく応用しだいでいろいろ使えます。 |
|
ディタクラスの作成 |
前回ファイル指定ダイアログを表示するエディタクラスを作成しました、今回はそれにイメージを描画する処理を追加します。
イメージを描画するには次のメソッドをオーバーライドします。
true を返して独自の描画処理をサポートしていることを知らせるメソッドです。
[VB]
Public Overrides Function GetPaintValueSupported( _
ByVal context As System.ComponentModel.ITypeDescriptorContext) As Boolean
[C#]
public override bool GetPaintValueSupported(
System.ComponentModel.ITypeDescriptorContext context){
プロパティグリッドにイメージを描画するメソッドです。
[VB]
Public Overrides Sub PaintValue( _
ByVal e As System.Drawing.Design.PaintValueEventArgs)
[C#]
public override void PaintValue(System.Drawing.Design.PaintValueEventArgs e){
|
VB[ImagePropertyEditor.vb] |
Imports System.Windows.Forms.Design
Public Class ImagePropertyEditor
Inherits System.Drawing.Design.UITypeEditor
' ファイルオープンダイアログ
Private _editorUi As OpenFileDialog = Nothing
' 編集スタイルの取得
Public Overrides Function GetEditStyle( _
ByVal context As System.ComponentModel.ITypeDescriptorContext) _
As System.Drawing.Design.UITypeEditorEditStyle
' モーダルウィンドウスタイル
Return System.Drawing.Design.UITypeEditorEditStyle.Modal
End Function
' 値の編集
Public Overrides Function EditValue( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal provider As System.IServiceProvider, _
ByVal value As Object) As Object
' エディタサービスの取得
Dim editservice As IWindowsFormsEditorService = _
provider.GetService(GetType(IWindowsFormsEditorService))
' ファイルオープンダイアログの作成
If _editorUi Is Nothing Then
_editorUi = New OpenFileDialog()
' ダイアログの設定
_editorUi.Filter = "Bitmap files (*.bmp)|*.bmp|Jpeg files (*.jpg)|*.jpg" & _
"|PNG files (*.png)|*.png|all files (*.*)|*.*"
_editorUi.CheckFileExists = True
End If
' ダイアログに値を設定
_editorUi.FileName = DirectCast(value, String)
' ファイルオープンダイアログ表示
If _editorUi.ShowDialog() = DialogResult.OK Then
Return _editorUi.FileName
Else
Return value
End If
End Function
' 独自の描画処理をサポートする
Public Overrides Function GetPaintValueSupported( _
ByVal context As System.ComponentModel.ITypeDescriptorContext) As Boolean
Return True
End Function
' イメージを描画する
Public Overrides Sub PaintValue( _
ByVal e As System.Drawing.Design.PaintValueEventArgs)
Dim value As String = DirectCast(e.Value, String)
If String.IsNullOrEmpty(value) OrElse Not System.IO.File.Exists(value) Then
' イメージが指定されていない場合
e.Graphics.FillRectangle(Brushes.White, e.Bounds)
Else
' イメージのロード
Using image As Image = image.FromFile(value)
' イメージの描画
e.Graphics.DrawImage(image, e.Bounds, _
New Rectangle(0, 0, image.Width, image.Height), GraphicsUnit.Pixel)
End Using
End If
End Sub
End Class
|
|
C#[ImagePropertyEditor.cs] |
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows.Forms;
using System.Windows.Forms.Design;
namespace PropatyGrid_CS
{
public class ImagePropertyEditor: System.Drawing.Design.UITypeEditor
{
// ファイルオープンダイアログ
private OpenFileDialog _editorUi = null;
// 編集スタイルの取得
public override System.Drawing.Design.UITypeEditorEditStyle GetEditStyle(
System.ComponentModel.ITypeDescriptorContext context)
{
// モーダルウィンドウスタイル
return System.Drawing.Design.UITypeEditorEditStyle.Modal;
}
// 値の編集
public override object EditValue(
System.ComponentModel.ITypeDescriptorContext context,
IServiceProvider provider,
object value)
{
// エディタサービスの取得
IWindowsFormsEditorService editservice =
(IWindowsFormsEditorService)provider.GetService(
typeof(IWindowsFormsEditorService));
// 編集用のユーザーインターフェイス作成
if (_editorUi == null)
{
_editorUi = new OpenFileDialog();
// ダイアログの設定
_editorUi.Filter = "Bitmap files (*.bmp)|*.bmp|Jpeg files (*.jpg)|*.jpg|" +
"PNG files (*.png)|*.png|all files (*.*)|*.*";
_editorUi.CheckFileExists = true;
}
// ダイアログに値を設定
_editorUi.FileName = value as string;
// ファイルオープンダイアログ表示
if (_editorUi.ShowDialog() == DialogResult.OK)
{
// 編集された値を返す
return _editorUi.FileName;
}
else
{
return value;
}
}
// 独自の描画処理をサポートする
public override bool GetPaintValueSupported(
System.ComponentModel.ITypeDescriptorContext context)
{
return true;
}
// イメージを描画する
public override void PaintValue(System.Drawing.Design.PaintValueEventArgs e)
{
string value = e.Value as string;
if (string.IsNullOrEmpty(value) || !System.IO.File.Exists(value))
{
// イメージが指定されていない場合
e.Graphics.FillRectangle(System.Drawing.Brushes.White, e.Bounds);
}
else
{
// イメージのロード
using (System.Drawing.Image image = System.Drawing.Image.FromFile(value))
{
e.Graphics.DrawImage(image, e.Bounds,
new System.Drawing.Rectangle(0, 0, image.Width, image.Height),
System.Drawing.GraphicsUnit.Pixel);
}
}
}
}
}
|
|
イメージファイルをプロパティにもつ Class4 は前回と同じで変更はありません |
VB[Class4.vb] |
Public Class Class4
' クラスメンバ変数
Private _fileName As String = String.Empty
' イメージプロパティ
<System.ComponentModel.Category("すらすら")> _
<System.ComponentModel.Description("イメージファイル名のプロパティ")> _
<System.ComponentModel.Editor(GetType(ImagePropertyEditor), _
GetType(System.Drawing.Design.UITypeEditor))> _
Public Property ImageFile() As String
Get
Return _fileName
End Get
Set(ByVal value As String)
_fileName = value
End Set
End Property
End Class
|
|
C#[Class4.cs] |
using System;
using System.Collections.Generic;
using System.Text;
namespace PropatyGrid_CS
{
class Class4
{
//クラスメンバ変数
private string _fileName = string.Empty;
// イメージプロパティ
[System.ComponentModel.Category("すらすら")]
[System.ComponentModel.Description("イメージファイル名のプロパティ")]
[System.ComponentModel.Editor(typeof(ImagePropertyEditor),
typeof(System.Drawing.Design.UITypeEditor))]
public string ImageFile
{
get { return _fileName; }
set { _fileName = value; }
}
}
}
|
|
|
※このページで紹介しているサンプルコードについて管理者は動作保障をいたしません※
※サンプルコードを使用する場合は、自己責任でお願いします※
|
【楽天 ランキング:パソコン・周辺機器 - パソコン周辺機器】
|
|
|
|
このサイトはフリーソフトのMerge HTMLで作成されています。
このサイトはリンクフリーです。
|
ページの先頭に戻る |
Copyright© 2010-2015 Jun.Shiozaki All rights reserved. |
|
|
|