アクセス数 累計:000,114,112 昨日:000,000,111 本日:000,000,135
|
|
|
|
【Amazon ランキング:本 - コンピュータ・IT】
|
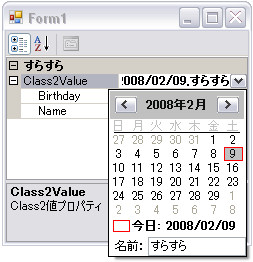 |
始めの章で Color型のプロパティをプロパティグリッドに設定していましたが、そのとき色を選択するダイアログが表示されていたことを覚えているでしょうか?
じつはこのダイアログ、自分で自由に作ることができるのです。プロパティ編集用のダイアログを表示させることによってユーザーインターフェイスを格段に向上させることができます。ここではその実装方法について説明します。 |
|
ユーザーインターフェイスの作成 |
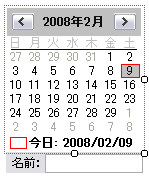 |
まずはプロパティの編集に使うユーザーインターフェイスを作成します。
プロジェクトの新しい項目の追加でユーザーコントロール「UserControl1」を作成してください。
使用するコントロールは次の3つです。
MonthCalender:[monthCalendar1]
Label:[label1]
TextBox:[textBox1]
それぞれのコントロールを左のイメージのように配置してください。
プロパティの値はすべてデフォルトを使用します。 |
|
VB[UserControl1.cs] |
'
Public Class UserControl1
' Class2 値プロパティ
Public Property Class2Value() As Class2
Get
Return New Class2(Me.monthCalendar1.SelectionStart, Me.textBox1.Text)
End Get
Set(ByVal value As Class2)
Me.monthCalendar1.SelectionStart = value.BirthDay
Me.textBox1.Text = value.Name
End Set
End Property
End Class
|
|
C#[UserControl1.cs] |
namespace PropatyGrid_CS
{
public partial class UserControl1 : UserControl
{
public UserControl1()
{
InitializeComponent();
}
// Class2 値プロパティ
public Class2 Class2Value
{
get
{
return new Class2(this.monthCalendar1.SelectionStart, this.textBox1.Text);
}
set
{
this.monthCalendar1.SelectionStart = value.Birthday;
this.textBox1.Text = value.Name;
}
}
}
}
|
|
エディタクラスの作成 |
ユーザーインターフェイス画面ができた後は、プロパティと画面をつなげるエディタクラスを作成します。
エディタクラスは [System.Drawing.Design.UITypeEditor]から派生させて次のメソッドをオーバーライドして記述します。
編集スタイルの編集モードを取得するメソッドです。
[VB]
Public Overrides Function GetEditStyle( _
ByVal context As System.ComponentModel.ITypeDescriptorContext) _
As System.Drawing.Design.UITypeEditorEditStyle
[C#]
public override System.Drawing.Design.UITypeEditorEditStyle GetEditStyle(
System.ComponentModel.ITypeDescriptorContext context){
編集画面を表示して変更された値を取得するメソッドです。
[VB]
Public Overrides Function EditValue( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal provider As System.IServiceProvider, _
ByVal value As Object) As Object
[C#]
public override object EditValue(
System.ComponentModel.ITypeDescriptorContext context,
IServiceProvider provider,
object value){
|
VB[Class2Editor.vb] |
Imports System.Windows.Forms.Design
Public Class Class2Editor
Inherits System.Drawing.Design.UITypeEditor
' 編集用ユーザーコントロール
Private _editorUi As UserControl1 = Nothing
' 編集スタイルの取得
Public Overrides Function GetEditStyle( _
ByVal context As System.ComponentModel.ITypeDescriptorContext) _
As System.Drawing.Design.UITypeEditorEditStyle
' ドロップダウンスタイル
Return System.Drawing.Design.UITypeEditorEditStyle.DropDown
End Function
' 値の編集
Public Overrides Function EditValue( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal provider As System.IServiceProvider, _
ByVal value As Object) As Object
' エディタサービスの取得
Dim editservice As IWindowsFormsEditorService = _
provider.GetService(GetType(IWindowsFormsEditorService))
' 編集用のユーザーインターフェイス作成
If _editorUi Is Nothing Then
_editorUi = New UserControl1
End If
' コントロールに値を設定
_editorUi.Class2Value = DirectCast(value, Class2)
' 編集用のコントロールを設定
editservice.DropDownControl(_editorUi)
' 編集された値を返す
Return _editorUi.Class2Value
End Function
End Class
|
|
C#[Class2Editor.cs] |
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows.Forms.Design;
namespace PropatyGrid_CS
{
public class Class2Editor:System.Drawing.Design.UITypeEditor
{
// 編集用ユーザーコントロール
private UserControl1 _editerUi = null;
// 編集用ユーザーコントロール
public override System.Drawing.Design.UITypeEditorEditStyle GetEditStyle(
System.ComponentModel.ITypeDescriptorContext context)
{
// ドロップダウンスタイル
return System.Drawing.Design.UITypeEditorEditStyle.DropDown;
}
// 値の編集
public override object EditValue(
System.ComponentModel.ITypeDescriptorContext context,
IServiceProvider provider,
object value)
{
// エディタサービスの取得
IWindowsFormsEditorService editservice =
(IWindowsFormsEditorService)provider.GetService(
typeof(IWindowsFormsEditorService));
// 編集用のユーザーインターフェイス作成
if (_editerUi == null)
{
_editerUi = new UserControl1();
}
// コントロールに値を設定
_editerUi.Class2Value = value as Class2;
// 編集用のコントロールを設定
editservice.DropDownControl(_editerUi);
// 編集された値を返す
return _editerUi.Class2Value;
}
}
}
|
|
Class2の方では System.ComponentModel.Editor属性で上で作成したエディタクラスとの関連付けを行います。
[VB]
<System.ComponentModel.Editor(GetType(Class2Editor), _ GetType(System.Drawing.Design.UITypeEditor))>
[C#]
[System.ComponentModel.Editor(typeof(Class2Editor), typeof(System.Drawing.Design.UITypeEditor ))]
|
VB[Class2.vb] |
<System.ComponentModel.Editor(GetType(Class2Editor), _
GetType(System.Drawing.Design.UITypeEditor))> _
<System.ComponentModel.TypeConverter(GetType(Class2PropertyConverter))> _
Public Class Class2
' クラスメンバ変数
Private _birthday As DateTime = Now
Private _name As String = "SuraSura"
' コンストラクタ
Public Sub New(ByVal birthday As DateTime, ByVal name As String)
' メンバ変数初期化
_birthday = birthday
_name = name
End Sub
' 誕生日プロパティ
<System.ComponentModel.Category("すらすら")> _
<System.ComponentModel.Description("誕生日")> _
Public Property BirthDay() As String
Get
Return _birthday
End Get
Set(ByVal value As String)
_birthday = value
End Set
End Property
' 名前プロパティ
<System.ComponentModel.Category("すらすら")> _
<System.ComponentModel.Description("名前")> _
Public Property Name() As String
Get
Return _name
End Get
Set(ByVal value As String)
_name = value
End Set
End Property
' 文字列の取得
Public Overrides Function ToString() As String
Return String.Format("{0:yyyy/MM/dd},{1}", _birthday, _name)
End Function
End Class
|
|
C#[Class2.cs] |
using System;
using System.Collections.Generic;
using System.Text;
namespace PropatyGrid_CS
{
[System.ComponentModel.Editor(typeof(Class2Editor),
typeof(System.Drawing.Design.UITypeEditor ))]
[System.ComponentModel.TypeConverter(typeof(Class2PropertyConverter))]
public class Class2
{
// クラスメンバ変数
private DateTime _birthday = DateTime.Now;
private string _name = "すらすら";
// コンストラクタ
public Class2(DateTime birthday, string name)
{
// メンバ変数初期化
_birthday = birthday;
_name = name;
}
// 誕生日プロパティ
public DateTime Birthday
{
get { return _birthday; }
set { _birthday = value; }
}
// 名前プロパティ
public string Name
{
get { return _name; }
set { _name = value; }
}
// 文字列の取得
public override string ToString()
{
return string.Format("{0:yyyy/MM/dd},{1}", _birthday, _name);
}
}
}
|
|
Class3 は前回と同じで変更はありません。 |
VB[Class3.vb] |
Public Class Class3
' クラスメンバ変数
Private _class2 As Class2 = New Class2()
' Class2値プロパティ
<System.ComponentModel.Category("すらすら")> _
<System.ComponentModel.Description("Class2 値のプロパティ")> _
Public Property Class2Value() As Class2
Get
Return _class2
End Get
Set(ByVal value As Class2)
_class2 = value
End Set
End Property
End Class
|
|
C#[Class3.cs] |
using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing;
namespace PropatyGrid_CS
{
public class Class3
{
// クラスメンバ変数
private Class2 _class2 = new Class2();
// Class2値プロパティ
[System.ComponentModel.Category("すらすら")]
[System.ComponentModel.Description("Class2値プロパティ")]
public Class2 Class2Value
{
get { return _class2; }
set { _class2 = value; }
}
}
}
|
|
|
※このページで紹介しているサンプルコードについて管理者は動作保障をいたしません※
※サンプルコードを使用する場合は、自己責任でお願いします※
|
【楽天 ランキング:フィギュア - アニメ・コミック】
|
|
|
|
このサイトはフリーソフトのMerge HTMLで作成されています。
このサイトはリンクフリーです。
|
ページの先頭に戻る |
Copyright© 2010-2015 Jun.Shiozaki All rights reserved. |
|
|
|