|
【Amazon ランキング:ゲーム - PS Vita】
|
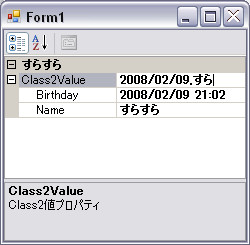 |
前回は独自クラスをプロパティグリッドに表示する方法を紹介しましたが、まだ完璧ではありません。
たとえば、Fomr オブジェクトの Location プロパティを思い浮かべてもらうとわかると思いますが階層を展開しなくてもプロパティを編集できるはずです。それはプロパティに入力されたテキストをPoint型 に変換して Location プロパティに設定しているためです。
このようなテキスト⇔オブジェクトの相互変換はコンバーターによって実現されています。ここでは先ほどの Class2 のコンバータークラスを作成してプロパティダイアログで編集できるようにしてみます。 |
|
コンバータークラスの作成 |
まずは、Class2 ⇔ String の相互変換を行うためのコンバータークラスの作成を行います。コンバータークラスは[ExpandableObjectConverter]から派生させたクラスに変換に必要なメソッドをオーバーライドして作成します。
[Class2 → String 変換に必要なメソッド]
コンバーターが指定された型変換に対応している場合に true を返すメソッドです。今回作成するコンバーターは型変換に対応しているので true
を返します。
[VB]
Public Overrides Function CanConvertTo( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal destinationType As System.Type) As Boolean
[C#]
public override bool CanConvertTo(
System.ComponentModel.ITypeDescriptorContext context,
Type destinationType){
実際に文字列にコンバート処理を行うメソッドです。変換した String値を戻り値で返します。
[VB]
Public Overrides Function ConvertTo( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal culture As System.Globalization.CultureInfo, _
ByVal value As Object, _
ByVal destinationType As System.Type) As Object
[C#]
public override object ConvertTo(
System.ComponentModel.ITypeDescriptorContext context,
System.Globalization.CultureInfo culture,
object value,
Type destinationType){
[String → Class2 変換に必要なメソッド]
コンバーターが文字列から指定された型への変換に対応している場合に true を返すメソッドです。今回作成するコンバーターは文字列からの型変換に対応させるので
true を返します。
[VB]
Public Overrides Function CanConvertFrom( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal sourceType As System.Type) As Boolean
[CS]
public override bool CanConvertFrom(
System.ComponentModel.ITypeDescriptorContext context,
Type sourceType){
実際にClass2にコンバート処理を行うメソッドです。変換した Class2値を戻り値で返します。
[VB]
Public Overrides Function ConvertFrom( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal culture As System.Globalization.CultureInfo, _
ByVal value As Object) As Object
[C#]
public override object ConvertFrom(
System.ComponentModel.ITypeDescriptorContext context,
System.Globalization.CultureInfo culture,
object value){
|
VB[Class2PropertyConverter.vb] |
' Class2 プロパティコンバータークラス
Public Class Class2PropertyConverter
Inherits System.ComponentModel.ExpandableObjectConverter
' オブジェクトをテキストにコンバートできるかどうかを返します
Public Overrides Function CanConvertTo( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal destinationType As System.Type) As Boolean
Return True
End Function
' オブジェクトをテキストにコンバートします。
Public Overrides Function ConvertTo( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal culture As System.Globalization.CultureInfo, _
ByVal value As Object, _
ByVal destinationType As System.Type) As Object
' Class2 ⇒String の変換が指定された場合
If destinationType Is GetType(System.String) AndAlso TypeOf value Is Class2 Then
Return DirectCast(value, Class2).ToString()
End If
Return MyBase.ConvertTo(context, culture, value, destinationType)
End Function
' テキストをオブジェクトにコンバートできるかどうかを返します
Public Overrides Function CanConvertFrom( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal sourceType As System.Type) As Boolean
Return True
End Function
' テキストをオブジェクトにコンバートします。
Public Overrides Function ConvertFrom( _
ByVal context As System.ComponentModel.ITypeDescriptorContext, _
ByVal culture As System.Globalization.CultureInfo, _
ByVal value As Object) As Object
' String ⇒Class2 の変換が指定された場合
If TypeOf value Is String Then
' value をカンマで区切る
Dim values() As String = DirectCast(value, String).Split(",")
Return New Class2(DateTime.Parse(values(0)), values(1))
End If
Return MyBase.ConvertFrom(context, culture, value)
End Function
End Class
|
|
C#[Class2PropertyConverter.cs] |
using System;
using System.Collections.Generic;
using System.Text;
namespace PropatyGrid_CS
{
// Class2 プロパティコンバータークラス
class Class2PropertyConverter:System.ComponentModel.ExpandableObjectConverter
{
// オブジェクトをテキストにコンバートできるかどうかを返します
public override bool CanConvertTo(
System.ComponentModel.ITypeDescriptorContext context,
Type destinationType)
{
return true;
}
// オブジェクトをテキストにコンバートします。
public override object ConvertTo(
System.ComponentModel.ITypeDescriptorContext context,
System.Globalization.CultureInfo culture,
object value,
Type destinationType)
{
// Class2 ⇒String の変換が指定された場合
if (destinationType == typeof(Class2) && value is Class2)
return (value as Class2).ToString();
return base.ConvertTo(context, culture, value, destinationType);
}
// テキストをオブジェクトにコンバートできるかどうかを返します
public override bool CanConvertFrom(
System.ComponentModel.ITypeDescriptorContext context,
Type sourceType)
{
return true;
}
// テキストをオブジェクトにコンバートします。
public override object ConvertFrom(
System.ComponentModel.ITypeDescriptorContext context,
System.Globalization.CultureInfo culture,
object value)
{
if (value is string)
{
string[] values = (value as string).Split(',');
return new Class2(DateTime.Parse(values[0]), values[1]);
}
return base.ConvertFrom(context, culture, value);
}
}
}
|
|
Class2の方は System.ComponentModel.TypeConverter を上で作成した[Class2PropertyConverter]に変更して、コンストラクタを追加します。
[VB]
<System.ComponentModel.TypeConverter(GetType(Class2PropertyConverter))>
[C#]
[System.ComponentModel.TypeConverter(typeof(Class2PropertyConverter))]
|
VB[Class2.vb] |
<System.ComponentModel.TypeConverter(GetType(Class2PropertyConverter))> _
Public Class Class2
' クラスメンバ変数
Private _birthday As DateTime = Now
Private _name As String = "SuraSura"
' コンストラクタ
Public Sub New(ByVal birthday As DateTime, ByVal name As String)
' メンバ変数初期化
_birthday = birthday
_name = name
End Sub
' 誕生日プロパティ
<System.ComponentModel.Category("すらすら")> _
<System.ComponentModel.Description("誕生日")> _
Public Property BirthDay() As String
Get
Return _birthday
End Get
Set(ByVal value As String)
_birthday = value
End Set
End Property
' 名前プロパティ
<System.ComponentModel.Category("すらすら")> _
<System.ComponentModel.Description("名前")> _
Public Property Name() As String
Get
Return _name
End Get
Set(ByVal value As String)
_name = value
End Set
End Property
' 文字列の取得
Public Overrides Function ToString() As String
Return String.Format("{0:yyyy/MM/dd},{1}", _birthday, _name)
End Function
End Class
|
|
C#[Class2.cs] |
using System;
using System.Collections.Generic;
using System.Text;
namespace PropatyGrid_CS
{
[System.ComponentModel.TypeConverter(typeof(Class2PropertyConverter))]
public class Class2
{
// クラスメンバ変数
private DateTime _birthday = DateTime.Now;
private string _name = "すらすら";
// コンストラクタ
public Class2(DateTime birthday, string name)
{
// メンバ変数初期化
_birthday = birthday;
_name = name;
}
// 誕生日プロパティ
public DateTime Birthday
{
get { return _birthday; }
set { _birthday = value; }
}
// 名前プロパティ
public string Name
{
get { return _name; }
set { _name = value; }
}
// 文字列の取得
public override string ToString()
{
return string.Format("{0:yyyy/MM/dd},{1}", _birthday, _name);
}
}
}
|
|
Class3 は前回と同じで変更はありません。 |
VB[Class3.vb] |
Public Class Class3
' クラスメンバ変数
Private _class2 As Class2 = New Class2()
' Class2値プロパティ
<System.ComponentModel.Category("すらすら")> _
<System.ComponentModel.Description("Class2 値のプロパティ")> _
Public Property Class2Value() As Class2
Get
Return _class2
End Get
Set(ByVal value As Class2)
_class2 = value
End Set
End Property
End Class
|
|
C#[Class3.cs] |
using System;
using System.Collections.Generic;
using System.Text;
using System.Drawing;
namespace PropatyGrid_CS
{
public class Class3
{
// クラスメンバ変数
private Class2 _class2 = new Class2();
// Class2値プロパティ
[System.ComponentModel.Category("すらすら")]
[System.ComponentModel.Description("Class2値プロパティ")]
public Class2 Class2Value
{
get { return _class2; }
set { _class2 = value; }
}
}
}
|
|
|
※このページで紹介しているサンプルコードについて管理者は動作保障をいたしません※
※サンプルコードを使用する場合は、自己責任でお願いします※
|
【楽天 ランキング:パソコン・周辺機器 - ネットワーク機器】
|
|
|
|
このサイトはフリーソフトのMerge HTMLで作成されています。
このサイトはリンクフリーです。
|
ページの先頭に戻る |
Copyright© 2010-2015 Jun.Shiozaki All rights reserved. |
|
|
|