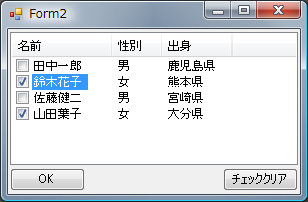 |
リストビューにはチェックボックスを表示することができます。
チェックボックスを表示するには CheckBoxes プロパティに True を指定します。 |
|
チェックされたアイテムの取得 |
チェックされたアイテムにはリストビューアイテムクラスの Checkd プロパティに Ture が設定されますので、Items コレクションからチェックされたアイテムを for 文を使って抽出します。
[VB]
For Each item As ListViewItem In Me.listView1.Items
If item.Checked Then
If txt.Length > 0 Then
txt.Append(",")
End If
End If
txt.Append(item.Text)
Next
[C#]
foreach( ListViewItem item in this.listView1.Items ){
if (item.Checked)
{
if (txt.Length > 0)
txt.Append(",");
txt.Append(item.Text);
}
}
|
フォームの作成 |
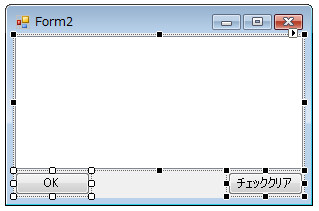 |
Form2 を作成して ListView、Button を貼り付けます
ListView:[listView1]
|
CheckBoxex |
True |
|
View |
Details |
Button:[Button1]
Button:[Button2]
|
|
VB[Form2.vb] |
Imports System.Text
Public Class Form2
Private Sub Form2_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
'リストビューに表示するデータ配列
Dim data(,) As String = New String(,) { _
{"田中一郎", "男", "鹿児島県"}, _
{"鈴木花子", "女", "熊本県"}, _
{"佐藤健二", "男", "宮崎県"}, _
{"山田葉子", "女", "大分県"}}
' ヘッダーの設定
Me.listView1.Columns.Add("名前", 100)
Me.listView1.Columns.Add("性別", 50)
Me.listView1.Columns.Add("出身", 70)
' リストビューのデータ追加
For row As Integer = 0 To 3
' リストビューアイテム作成
Dim item As ListViewItem = New ListViewItem(data(row, 0))
' サブアイテム追加
item.SubItems.Add(data(row, 1))
item.SubItems.Add(data(row, 2))
' アイテムをリストビューに追加
Me.listView1.Items.Add(item)
Next
End Sub
Private Sub button1_Click( _
ByVal sender As System.Object, ByVal e As System.EventArgs) Handles button1.Click
Dim txt As StringBuilder = New StringBuilder()
' チェックがついているアイテム取得
For Each item As ListViewItem In Me.listView1.Items
If item.Checked Then
If txt.Length > 0 Then
txt.Append(",")
End If
End If
txt.Append(item.Text)
Next
MessageBox.Show(String.Format("選択アイテム[{0}]", txt.ToString()))
End Sub
Private Sub Button2_Click( _
ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button2.Click
' チェックをはずす
For Each item As ListViewItem In Me.listView1.Items
If item.Checked Then
item.Checked = False
End If
Next
End Sub
End Class
|
|
C#[Form2.cs] |
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace ListViewCS
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void Form2_Load(object sender, EventArgs e)
{
// リストビューに表示するデータ配列
string[,] data = new string[,]{
{"田中一郎","男","鹿児島県"},
{"鈴木花子","女","熊本県"},
{"佐藤健二","男","宮崎県"},
{"山田葉子","女","大分県"}};
// ヘッダーの設定
this.listView1.Columns.Add("名前", 100);
this.listView1.Columns.Add("性別", 50);
this.listView1.Columns.Add("出身", 70);
// リストビューのデータ追加
for (int row = 0; row < 4; row++)
{
// リストビューアイテム作成
ListViewItem item = new ListViewItem(data[row, 0]);
// サブアイテム追加
item.SubItems.Add(data[row, 1]);
item.SubItems.Add(data[row, 2]);
// アイテムをリストビューに追加
this.listView1.Items.Add(item);
}
}
private void button1_Click(object sender, EventArgs e)
{
StringBuilder txt = new StringBuilder();
// チェックがついているアイテム取得
foreach( ListViewItem item in this.listView1.Items ){
if (item.Checked)
{
if (txt.Length > 0)
txt.Append(",");
txt.Append(item.Text);
}
}
MessageBox.Show( string.Format("選択アイテム[{0}]",txt.ToString()));
}
private void Button2_Click(object sender, EventArgs e)
{
// チェックをはずす
foreach (ListViewItem item in this.listView1.Items)
{
if (item.Checked)
item.Checked = false;
}
}
}
}
|
|
|